Software localization
Creating Multilingual PHP Apps with Fat-Free Framework
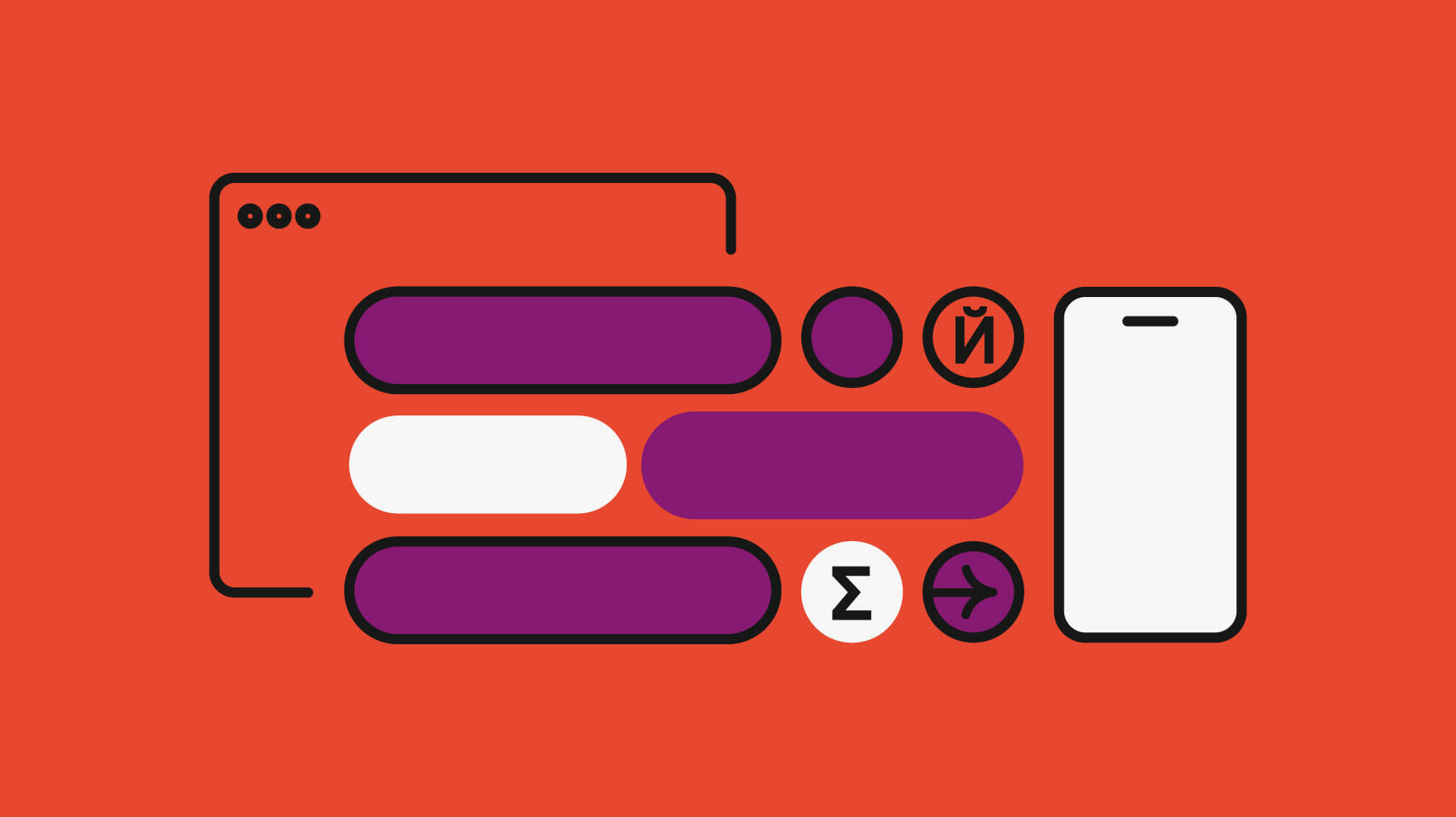
Background
I recently read a post about an amazing project done with Fat-Free Framework (or simply, “F3”), a PHP micro framework. So I gave it a try to find out how it handles localization.
Getting Started
The good new is: F3 has built-in support for multilingual apps. In this example, I will create a project with the following basic directory structure:
index.php
lib/ (F3 base)
app/ (application files)
dict/ (create this directory!)
controllers/
models/
views/
F3 automatically detects language files in the ‘dict’ directory. Even better: F3 detects a users language based on the HTTP Accept-Language header coming from the browser and uses the right language file.
Here is my minimal app code in index.php:
<?php $f3 = require('lib/base.php'); $f3->set('LOCALES','app/dict/'); $f3->route('GET /', function($f3) { $template = new Template; echo $template->render('app/views/index.html'); } ); $f3->run(); ?>
Creating Language Files
For this example I want to use two languages: English and German.
For English, I create a new file (en.php) in the ‘dict’ directory.
<?php return array( 'hello' => 'Hello', 'world' => 'World', ); ?>
For German, I create de.php:
<?php return array( 'hello' => 'Hallo', 'world' => 'Welt', ); ?>
It is also possible to create language files for language variants, such as en-US, en-GB or de-DE, de-AT. If a key doesn’t exist in a variant, F3 will search for it in the root language and at last, always fall back to the default English ‘en.php’ language file.
Using Language Files In F3 Templates
Loading strings from the language files in to your templates is easy, here is my index.html template:
<h1>{{ @hello }}</h1> <h2>{{ @world }}</h2>
Use Translations In Your Code
I can also access translated strings from within the app code:
$hello = $f3->get('hello');
Advanced Translations
Some of the more advanced localization techniques that F3 supports are placeholders and pluralization.
Placeholders
In the language file:
<?php return array( 'hello' => 'Hello', 'new_messages' => 'You have {0} new messages', ); ?>
In the app code:
echo $f3->format($f3->get('new_messages'), 42); // Output: "You have 42 new messages"
Pluralization
The plural syntax is a bit tricky, but very helpful. Here is what the language file looks like:
<?php return array( 'hello' => 'Hello', 'orders' => '{0, plural,'. 'zero {No new order},'. 'one {One new order},'. 'other {You have # new orders}'. '}' ); ?>
Quantities recognized by F3 are ‘zero’, ‘one’, ‘two’ and ‘other’.
Usage in the app code:
echo $f3->format($f3->get('orders'), 0); // Output: "No new order"
Testing
As mentioned earlier, F3 automatically serves the right language. For testing purposes, if you don’t want to mess around in your browser language settings, you can force F3 to use a specific language file:
// index.php $f3->set('LOCALES','app/dict/'); $f3->set('LANGUAGE','de');
Managing Translations
Working with .php language files can be cumbersome: new keys must be added to each language file separately, the same goes for updating keys.
Phrase is a translation management tool that addresses some of the issues. It also features a powerful In-Context Editor (Demo), making the process of translating web apps more convenient.
Integrating the Phrase In-Context Editor in your F3 apps is easy. Get our special language file and save it in your ‘dict’ directory. Give it a two letter name that isn’t used, for example ‘xx.php’. In your app, force the use of the Phrase language file that exposes your keys to the Phrase editor:
<?php $f3 = require('lib/base.php'); $f3->set('LOCALES','app/dict/'); $f3->set('LANGUAGE','xx'); $f3->route('GET /', function($f3) { $template = new Template; echo $template->render('app/views/index.html'); } ); $f3->run(); ?>
Then include the JavaScript snippet in your templates:
<script> var phrase_auth_token = 'YOUR_PHRASEAPP_TOKEN'; (function() { var phraseapp = document.createElement('script'); phraseapp.type = 'text/javascript'; phraseapp.async = true; phraseapp.src = ['https://', 'phraseapp.com/assets/phrase/0.1/app.js?', new Date().getTime()].join(''); var s = document.getElementsByTagName('script')[0]; s.parentNode.insertBefore(phraseapp, s); })(); </script>
That’s it - you can now translate your site using the Phrase In-Context Editor. Phrase also lets you order professional translations. “Se habla Español” for your app? With Phrase, it’s just one click away.
Further Reading
Be sure to subscribe and receive all updates from the Phrase blog straight to your inbox. You’ll receive localization best practices, about cultural aspects of breaking into new markets, guides and tutorials for optimizing software translation and other industry insights and information. Don’t miss out!